ScratchLink
ScratchLink is an educational WIFI robotics and coding system suitable for Primary & Up with WIFI browser based coding with live code execution and real time sensor data. Integrated Gamepad functionality for extra fun. Code with visual ScratchLink Blocks or our Javascript Coding Editor. Compatible with all devices (PC, Mac, iPad, iPhone & Android) with no software or updates to install. Three levels of inbuilt robotics challenges plus our Virtual Robotics Simulator.
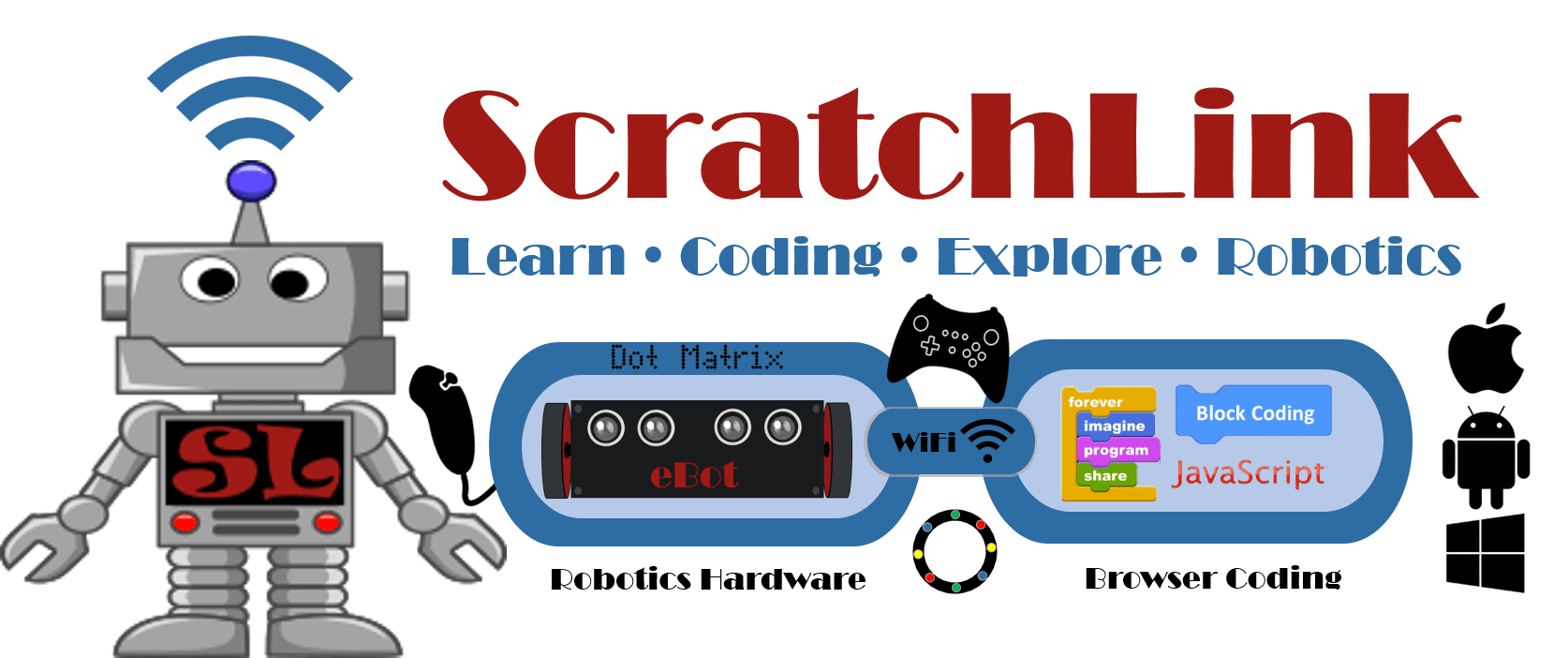
eBots
We design and manufacture a range of robust educational robotics i.e. eBots, including mobile robots, cranes and sorting conveyers etc. Our mobile robots have large rechargeable batteries with on board USB charging that provide a full school day of use. Options include 2WD, Tank and Omni drive plus tough all metal crane arms, RFID, claw proximity sensors and WIFI camera. Standard on all our mobile robots (except Junior) are 2x line sensors, 2x ultrasonic range finders, colour sensor, 2x colour LED rings, 16×16 Dot Matrix display.

JS4eBot Introduction
JS4eBot is the JavaScript based coding language that is used in our Coding Editor to control our educational robotics hardware i.e. eBots.
Syntax Overview

Commands are suffixed with “Cmd” and must go last on the line. Commands may or may not take parameters.
Parameters are inputs for Commands and Specifiers and are contained in brackets i.e. “(parameter)”.
Starting commands with “eBot.” is optional. If you start commands with “eBot.” then you will get full “intellisence” suggestions for all the commands but your code is longer. If you already know the commands you can omit “eBot.” at the start.
Code Comments are denoted with a double forward slash “//” at the beginning of the line which means that line of code is ignored and serves as a comment only. It is good practice to explain your code with comments liberally.
eBot.led.colourWordCmd('green')
// or the following works too
led.colourWordCmd('green')
Specifiers define hardware aspects that the Command should apply eg. which LED ring or which Pixel etc. If no specifier is provided then the Command will apply to all hardware eg. all LED pixels on all LED rings. Specifiers must go before the command itself i.e. that which ends in “Cmd”.
// specifiers must go first then Cmd last
led.pixelRange(1,5).ring('LHS').colorRGBCmd(50,50,50)
// but the following is incorrect sytax and will not run
led.colorRGBCmd(50,50,50).pixelRange(1,5).ring('LHS')
Quotes are used for “String” variables and parameters. You can use either double or single quotes.
Example 1: Turn all pixels red on all LED rings.
led.colorWordCmd("red")
// OR
eBot.led.colorWordCmd("red")
Example 2: Turn pixels 1-5 red on just the left hand side LED ring.
led.pixelRange(1,5).ring('LHS').colorRGBCmd(50,50,50)
// OR
eBot.led.pixelRange(1,5).ring('LHS').colorRGBCmd(50,50,50)
JS Console
The JS Console is a component of Coding Editor.
Errors that occur when the code is run are shown in the JS Console along with the line number as shown below.
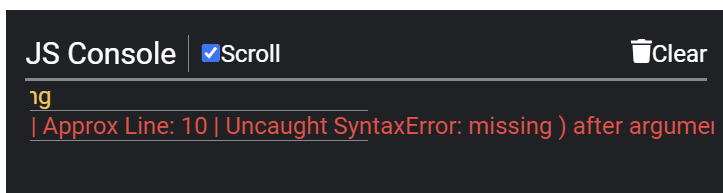
The JS Console can also be used for debugging e.g. shown below are console logs each time the ball hits and misses. The syntax for console log is:
console.log('Your Text')
// to log variable values
console.log(myVariable)
Reserved Words
JavaScript Reserved Words
The following are “JavaScript Reserved” words and also cannot be used as variables, labels or function names.
abstract | arguments | await | boolean |
break | byte | case | catch |
char | class | const | continue |
debugger | default | delete | do |
double | else | enum | eval |
export | extends | false | final |
finally | float | for | function |
goto | if | implements | import |
in | instanceof | int | interface |
let | long | native | new |
null | package | private | protected |
public | return | short | static |
super | switch | synchronized | this |
throw | throws | transient | true |
try | typeof | var | void |
volatile | while | with | yield |
JS4eBot Reserved Words
JSScratchLink | eBot | wheels |
led | matrix | crane |
eventsEbot | eventsGamepad1 | eventsGamepad2 |
control | config | runCodeOnStart |
started | ended | Button |
Supported GamePads
JS4Scratch supports 5 GamePads which can be purchased in our store or use your own Xbox wired or T3 BLE GamePad. We also manufacture two GamePads that utilise the Wii Nunchuk and Wii Classic Controller. You can either use a single GamePad or two of the same type at once. They are automatically detected when connected to our system.
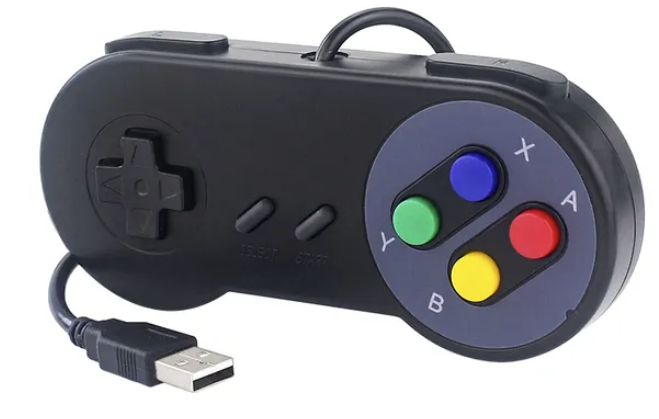
Retro USB wired GamePad. Simple USB wired controller. Suitable for Windows PC USB port. Our coding systems have been designed to work with this gamepad and supports two identical gamepads simultaneously for 2 player action.
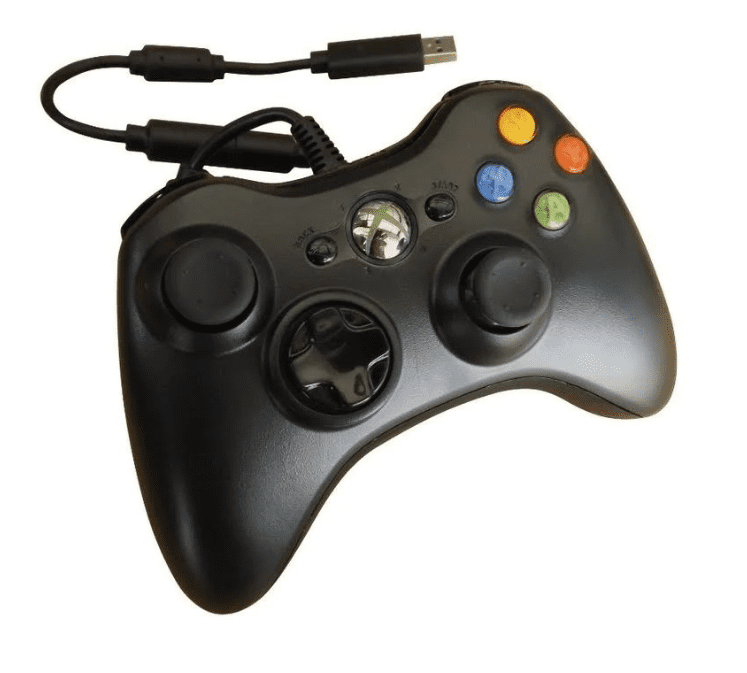
USB wired GamePad (xBox). Based on the acclaimed design of the Xbox Controller. Suitable for Windows PC USB port. Our coding systems have been designed to work with this gamepad and supports two identical gamepads simultaneously for 2 player action. Both the USB wired and T3(BLE) wireless GamePads have the same inputs i.e. 2 x Thumbsticks, D-pad, 6 x top buttons and 4 x front triggers.
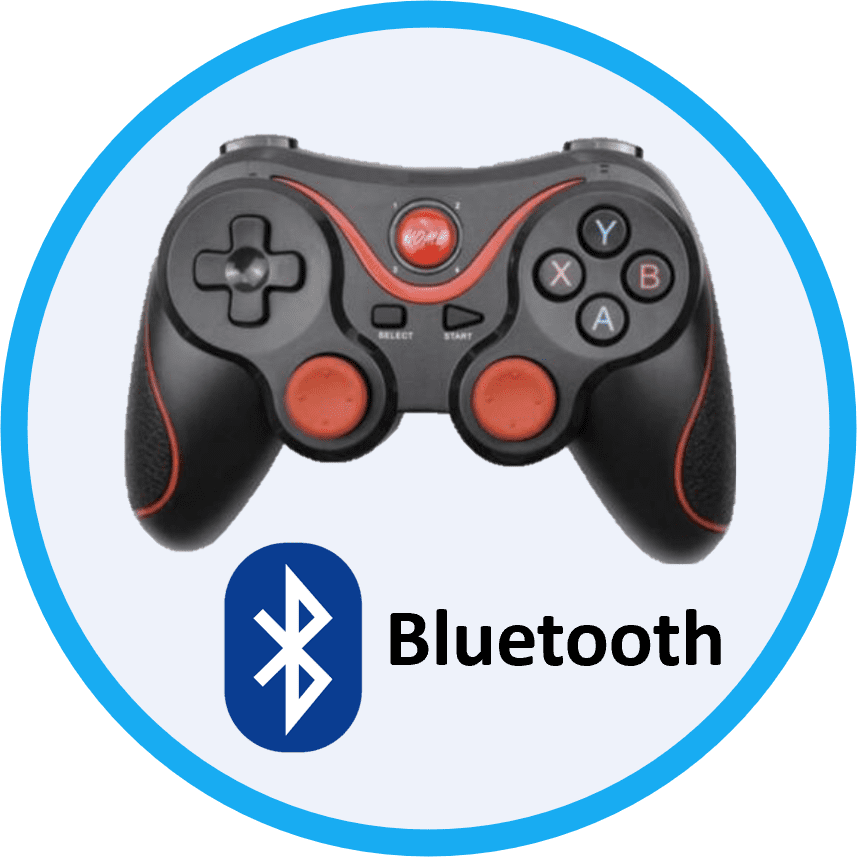
T3 Wireless (BLE) GamePad. Based on the acclaimed design of the Xbox Controller. Suitable for Windows, MAC, Android and IOS. Our coding systems have been designed to work with this gamepad and supports two identical gamepads simultaneously for 2 player action. Both the USB wired and T3(BLE) wireless GamePads have the same inputs i.e. 2 x Thumbsticks, D-pad, 6 x top buttons and 4 x front triggers.
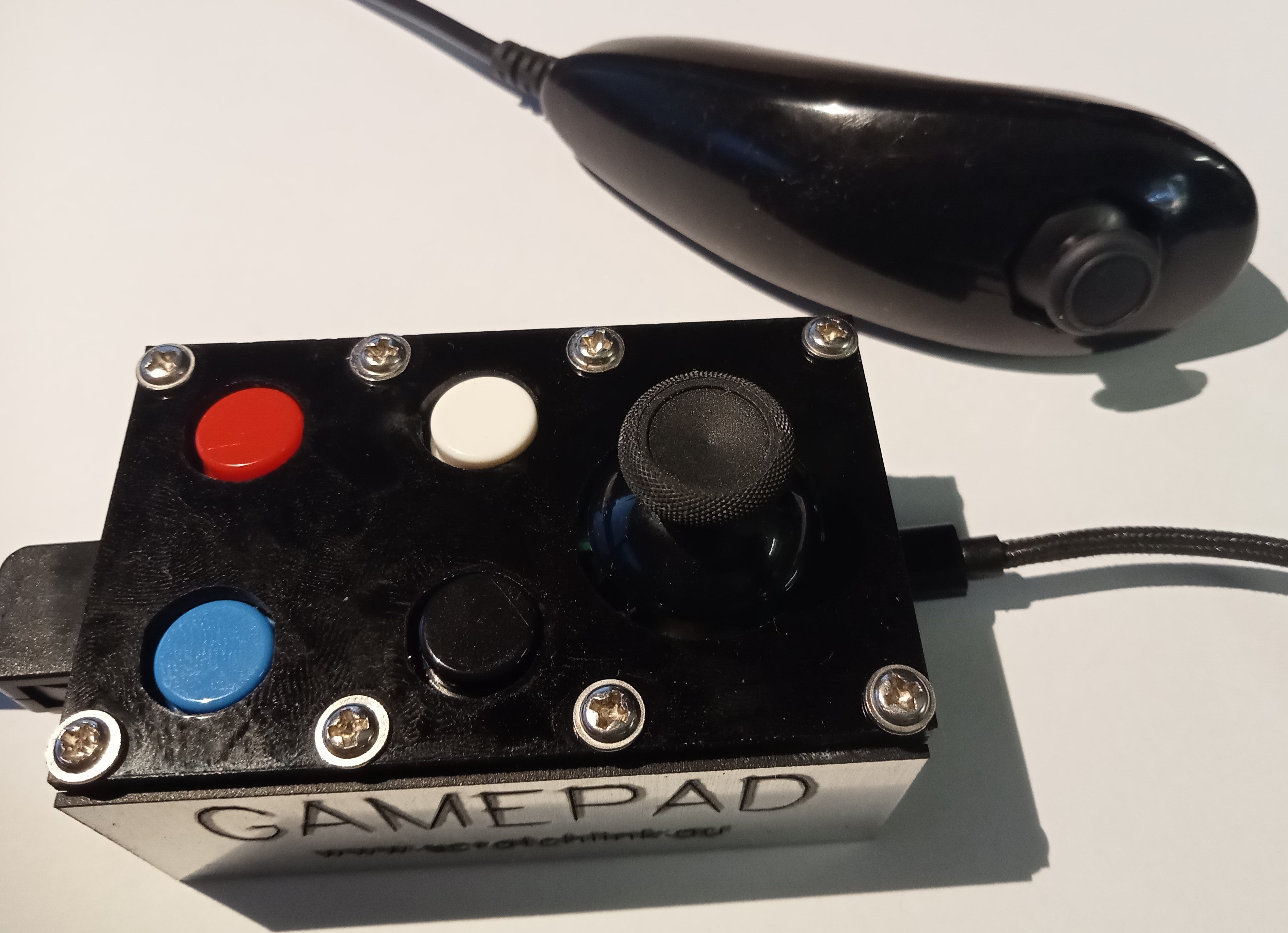
The ScratchLink NunChuck GamePad is a PC USB wired GamePad. It is two gamepads in one comprising our own design central module with connected Wii NunChuk controller. So two player action using this single GamePad. Our coding systems have been designed to work with this gamepad and supports two identical gamepads simultaneously.
Central Gamepad Module: 1 x Thumbstick with button & 4 x buttons
Nunchuck: 1 x Thumbstick + 2 x front triggers + X and Y Gyro
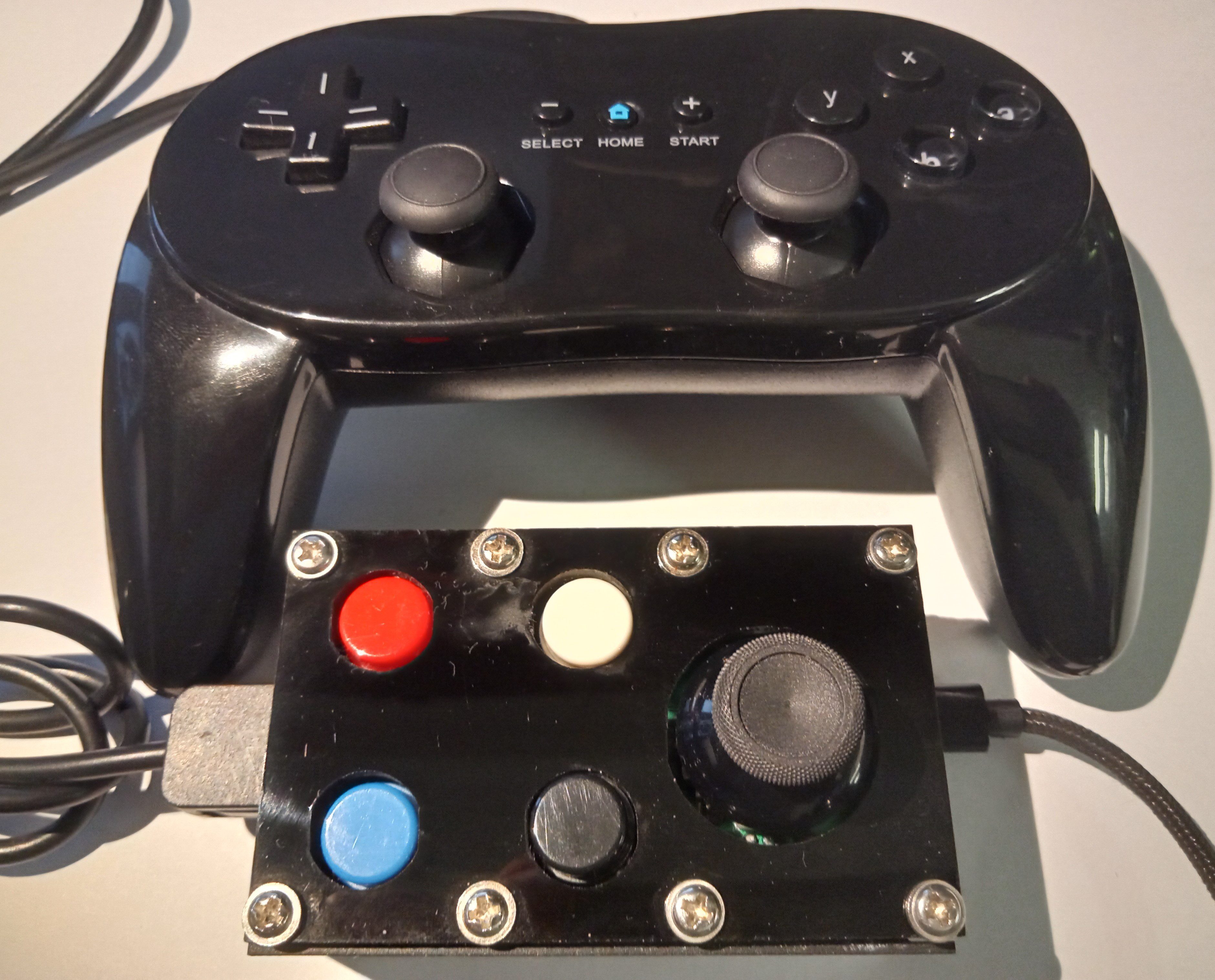
The ScratchLink Classic Controller GamePad is a PC USB wired GamePad. It is two gamepads in one comprising our own design central module with connected Wii Classic Controller. So two player action using this single GamePad. Our coding systems have been designed to work with this gamepad and supports two identical gamepads simultaneously.
Central Gamepad Module: 1 x Thumbstick with push button + 4 x buttons
Attached Classic Controller: 2 x Thumbsticks + 4 x front triggers + D-pad + 7 x top buttons.