When Flag
whenFlag(function () {
/* your code */
})
The whenFlag() event will run the code in the whenFlag() function after the “Flag” is clicked by the user. Note that you don’t have to put your code in a whenFlag() event function, in which case the code will start immediately without any user input.
// Example: whenFlag()
exampleSprite_sprite.whenFlag(function () {
this.say ('Code has started, but I am not in any loop')
})
// Example: No whenFlag()
exampleSprite_sprite.say ('Code has started, but I am not in any loop')
When Key Pressed
whenKeyPressed('key',function (){
/* your code */
}
// Example: whenKeyPressed()
exampleSprite_sprite.whenFlag(function () {
this.say ('Waiting for you to press the space bar key')
stage.whenKeyPressed('space',function (){
exampleSprite_sprite.say('Well done- you just pressed the space bar')
stage.wait(1)
exampleSprite_sprite.say ('Waiting for you to press the space bar key again')
})
})
You can also use this site to find the key code of your desired key or look them up yourself and use the keycode instead of the text string of that key. For example, you could replace ‘space’ with it’s keycode 32 but use 32 not ’32’.
// Example: whenKeyPressed()
exampleSprite_sprite.whenFlag(function () {
this.say ('Waiting for you to press the space bar key')
stage.whenKeyPressed(32,function (){
exampleSprite_sprite.say('Well done- you just pressed the space bar')
stage.wait(1)
exampleSprite_sprite.say ('Waiting for you to press the space bar key again')
})
})
When This Sprite Clicked
example_Sprite_sprite.whenClicked(function (){
/*your code*/
})
// Example: whenClicked
example_Sprite_sprite.whenFlag(function () {
example_Sprite_sprite.show()
example_Sprite_sprite.whenClicked(function (){
this.say('You just clicked me')
})
})
When I receive Message
whenReceiveMessage('message1', (function (){
/*your code*/
}))
// Example: whenReceiveMessage
stage.whenClicked(function (){
stage.broadcastMessage('move')
})
example_Sprite_sprite.show()
example_Sprite_sprite.say('click the stage to move me')
example_Sprite_sprite.whenReceiveMessage('move', (function (){
this.sayWait('You just clicked me',1)
this.move(20)
}))
New Message
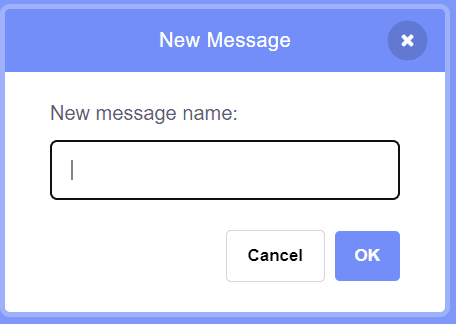
broadcastMessage('message_name')
Note that there is no specific “New Message” script, rather you simply broadcast the message directly without having to create a new message first as shown below.
// Example: broadcastMessage
stage.whenClicked(function (){
stage.broadcastMessage('move')
})
example_Sprite_sprite.show()
example_Sprite_sprite.say('click the stage to move me')
example_Sprite_sprite.whenReceiveMessage('move', (function (){
this.sayWait('You just clicked me',1)
this.move(20)
}))
Broadcast Message
broadcastMessage('message_name')
// Example: broadcastMessage
stage.whenClicked(function (){
stage.broadcastMessage('move')
})
example_Sprite_sprite.show()
example_Sprite_sprite.say('click the stage to move me')
example_Sprite_sprite.whenReceiveMessage('move', (function (){
this.sayWait('You just clicked me',1)
this.move(20)
}))